【python】横の数値との計算(ピラミッド計算など) NumPy
ピラミッド計算をpythonでやってみます。
ピラミッド計算とはどういうものかというと、下画像のようなピラミッド型の枠内に計算の答えを書いていき頂点の数値を求めるものです。今回は計算結果の下2桁を出力するプログラムを作成しています。
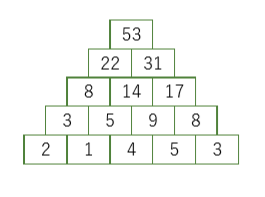
最初に思いつくのはfor 構文だと思いますのでfor 構文で書いてみます。
for 構文
# coding: utf-8
def get_score1(word):
list=[]
for num in word:
list.append(int(num))
# ここで隣同士を計算した新しい配列を作成し、データ数が1つになったら出力する
while len(list)>1:
list2=[]
for i in range(len(list)-1):
list2.append((list[i]+list[i+1])%100)
list = list2
return list[0]
input_line = input() #'1 2 3 4 5'
word = input_line.split()
print(get_score1(word))
桁数が多くなければ問題なく実行できます。が、桁数が多くなると実行時間が増えてしまいます
そこで、NumPyを使用します。
NumPy
# coding: utf-8
import numpy as np
def get_score2(word):
list=[]
for num in word:
list.append(int(num))
n1 = np.array(list)
# 配列のデータ数が1つになったら出力
while True:
n1 = (n1[1:]+n1[:-1])%100
if len(n1)==1:
break
return n1[0]
input_line = input() #'1 2 3 4 5'
word = input_line.split()
print(get_score12(word))
もっと最適化できると思います。
ご参考になれば幸いです!!
ディスカッション
コメント一覧
まだ、コメントがありません