【GAS】How to get operational data for TikTok ads
This is how to get operational data for TikTok ads using GAS (Google Apps Script). The official documentation included PHP and Python code, but there was no information on how to use GAS, so we put it together.
Create a TikTok Developer Account as a prerequisite.
This article was adapted from here.
TikTok Marketing API docs
https://ads.tiktok.com/marketing_api/docs
目次
Get APPID in TikTok Developer
https://ads.tiktok.com/marketing_api/apps/
Fill in the required information to create an APPID.
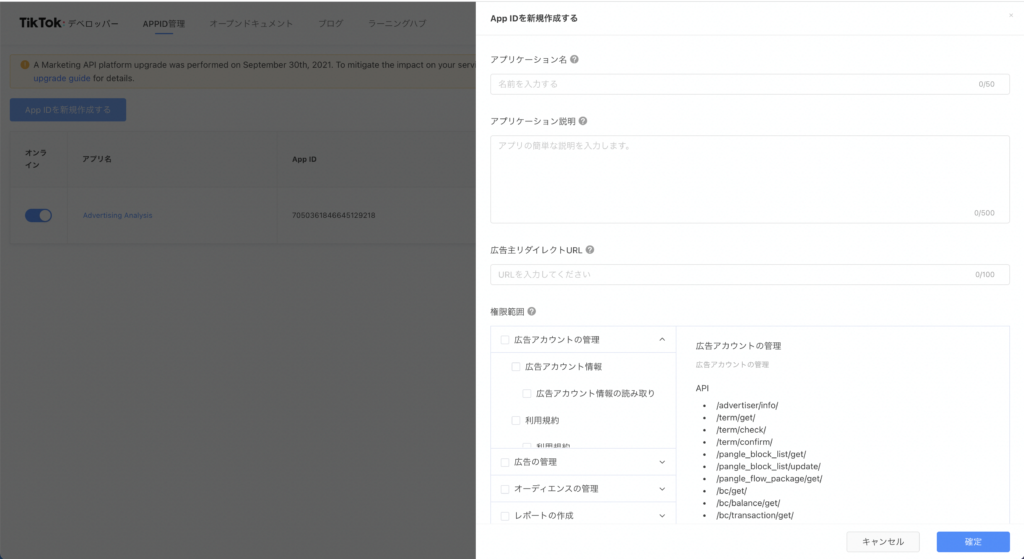
Obtain the advertiser’s auth_code
The auth_code is valid for 10 minutes and can only be used once. When the auth_code expires, you must start over and perform the authentication procedure again.
Click on the name of the application you created and copy and paste the “Advertiser authorization URL" to access it.
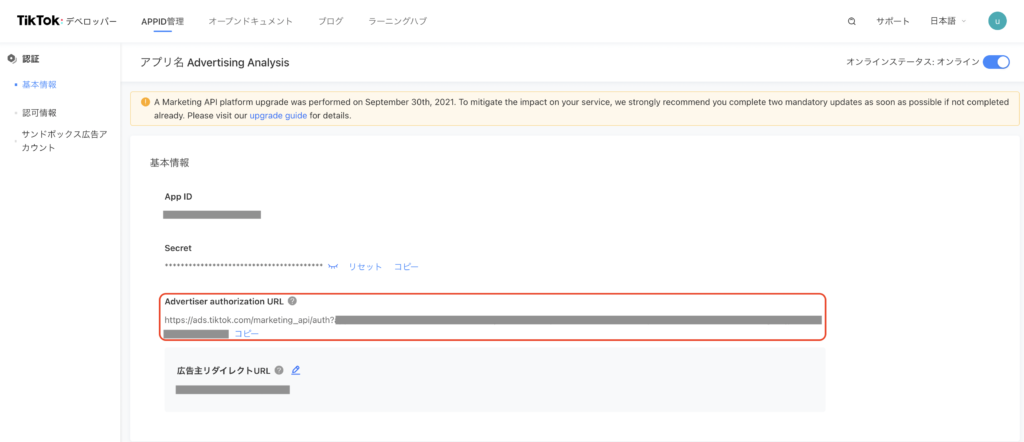
認証画面が出るので認証をすると、「広告主リダイレクトURL 」にリダイレクトされます。
After authentication, you will be redirected to the “Advertiser Redirect URL".
Generate long-term access token
GAS is used to obtain a long-term access token for generation. The code is as follows
function getAuthCode() {
const SECRET = APP_SECRET
const APP_ID = "*********"
const AUTH_CODE = "**********"
const PATH = "https://business-api.tiktok.com/open_api/v1.2/oauth2/access_token/";
let params = {
secret: SECRET,
app_id: APP_ID,
auth_code: AUTH_CODE,
};
let options = {
'method': 'post',
'contentType': 'application/json',
'payload': JSON.stringify(params)
};
let response = UrlFetchApp.fetch(PATH, options).getContentText();
console.log(response)
}
APP_SECRET : SECRET in APP Basic Information
APP_ID : ID of the created APP
AUTH_CODE : Code you have just obtained
After executing the above, an access token will be issued with the following text in the log screen.
{
"message": "OK",
"code": 0,
"data": {
"access_token": "xxxxxxxxxxxxx",
"scope": [
4
],
"advertiser_ids": [
1234,
1234
]
},
"request_id": "2020042715295501023125104093250"
}
Obtain operational data
The code to obtain operational data is shown below.
class TikTokADS{
constructor(op){
this.PATH = "https://ads.tiktok.com/open_api/v1.2/reports/campaign/get/";
this.ACCESS_TOKEN = op['ACCESS_TOKEN']
this.getStat = op['getStat']
this.advertiser_id = op['advertiser_id']
}
getAdsData() {
let option = {
primary_status : 'STATUS_ALL',
start_date : '2021-12-01',
end_date : '2021-12-31',
advertiser_id : this.advertiser_id,
fields : JSON.stringify(this.getStat),
group_by : JSON.stringify(['STAT_GROUP_BY_FIELD_STAT_TIME', 'STAT_GROUP_BY_FIELD_ID']),
time_granularity : 'STAT_TIME_GRANULARITY_DAILY', //'STAT_TIME_GRANULARITY_HOURLY'
page : 1,
page_size : 100,
}
let params
for (let key in option) {
params += key + "=" + option[key] + "&"
}
let url = encodeURI(this.PATH + "?" + params)
const httpResponse = UrlFetchApp.fetch(url, {
method: 'get',
muteHttpExceptions: true,
headers: {
'Access-Token': this.ACCESS_TOKEN,
},
})
.getContentText();
let res = JSON.parse(httpResponse)
let adsData = res["data"]["list"]
let result = [['stat_datetime','campaign_id','campaign_name',...this.getStat]]
for (let elm of adsData) {
let temp = []
temp.push(new Date(elm['stat_datetime']))
temp.push(String(elm['campaign_id']))
temp.push(elm['campaign_name'])
for (let stat of this.getStat) {
temp.push(elm[stat])
}
result.push(temp)
}
result = result.sort((a, b) => a[0] - b[0])
return result
}
}
function main(){
let Tk = new TikTokADS({
ACCESS_TOKEN :"*******************************",,
getStat :['campaign_name', 'stat_cost', 'show_cnt', 'click_cnt', 'convert_cnt', 'time_attr_view'], //取得したいデータを設定する
advertiser_id :"*************************",
})
let result = Tk.getAdsData()
const setSheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName('出力したいシート名')
setSheet.getRange(1, 1, result.length, result[0].length).setValues(result)
}
Executing the above will output data to the sheet.
Discussion
New Comments
No comments yet. Be the first one!