[GAS] How to get operational data of Yahoo Ads into a spreadsheet by yourself (v7 compatible)
Updated 2/28/2022 to v7.
This is a way to get operational data such as impressions and conversions of Yahoo ads into a spreadsheet by yourself. In this case, GAS (GoogleAppsScript) will be used.
For more information, please click here.
https://ads-developers.yahoo.co.jp/developercenter/ja/yahoo-ads-api/
Other Advertising Media
目次
Register Yahoo Ad Application
Ads API Management Tool and log in with the Yahoo! Business ID with which you are managing your ads.
Register an application.
Fill in the required information on the registration screen and select confirm. Enter “oob" for the redirect URI.
After registration is complete, the application information will be displayed as shown below, so note the Client ID and Client Secret.
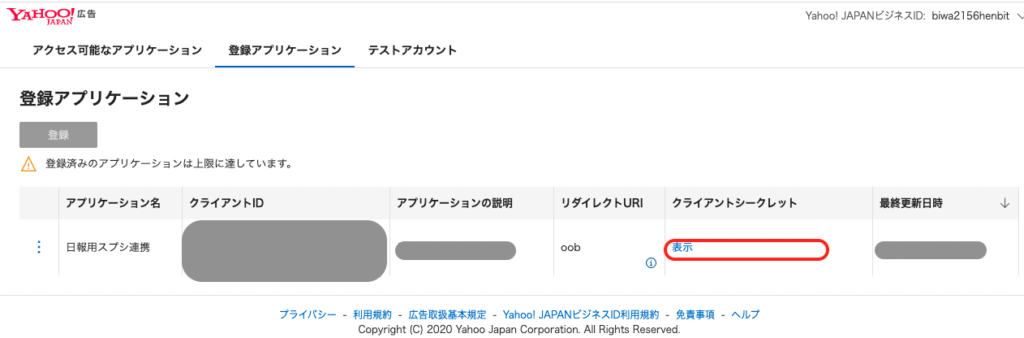
Obtaining an Authentication Code
Create the following URL
-CLIENT_ID: Client ID noted at the beginning of this document
-REDIRECT_URI: URL set in the application registration
-THIS_VALUE_SHOULD_BE_UNIQUE_PER_REQUEST: Arbitrary string (*Random number is OK)
https://biz-oauth.yahoo.co.jp/oauth/v1/authorize?response_type=code
&client_id=CLIENT_ID
&redirect_uri=REDIRECT_URI
&scope=yahooads
&state=THIS_VALUE_SHOULD_BE_UNIQUE_PER_REQUEST
Access the above with a browser and approve the access permission, and an authorization code will be displayed.
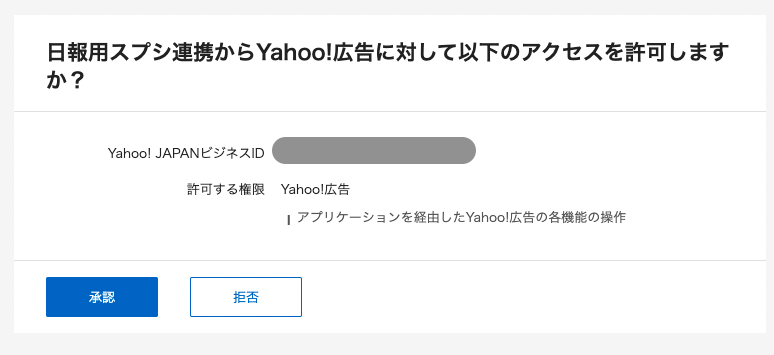
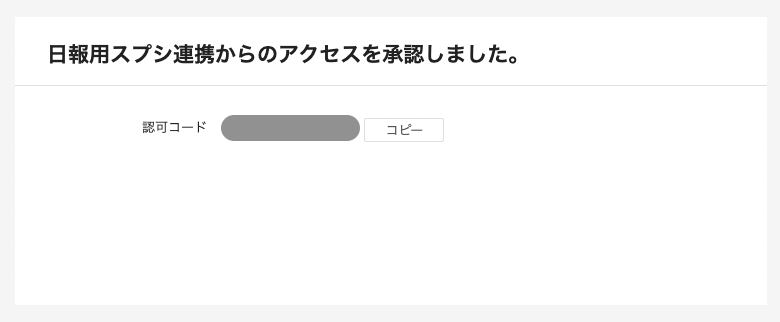
https://developer.yahoo.co.jp/yconnect/v1/server_app/explicit/authorization.html
Obtaining access and refresh tokens
Execute the following request from a terminal, etc.
-CLIENT_ID: Client ID that you wrote down at the beginning of this document
-CLIENT_SECRET: The client secret you wrote down at the beginning of this document.
-REDIRECT_URI: URL set in the application registration
-AUTH_CODE: Authorization code obtained earlier
curl -X GET
https://biz-oauth.yahoo.co.jp/oauth/v1/token?grant_type=authorization_code
&client_id=CLIENT_ID
&client_secret=CLIENT_SECRET
&redirect_uri=REDIRECT_URI
&code=AUTH_CODE
The access token and refresh token are returned as shown below, and the values of each are written down.
It can also be accessed with a browser.
Since the access token expires in one hour, a new access token must be issued each time using a refresh token.
Creating Spreadsheets and GAS
spreadsheet and create a “db" sheet.
Create a script from “Tools" -> “Scripts". The code is as follows
function main() {
// シート定義
const ss = SpreadsheetApp.getActiveSpreadsheet().getSheetByName('db');
// 各種設定
const clientId = "****"; // クライアントID
const clientSecret = "****"; // クライアントシークレット
const refreshToken = "****"; // リフレッシュトークン
const accountId = "****"; // 広告アカウントID
// Access token acquisition
const loginResponse = yahooAdLogin(clientId, clientSecret, refreshToken);
const accessToken = loginResponse.access_token;
// Create a YAHOO ad report and get Job's ID
const reportName = "test";
const createReportResponse = yahooAdCreateReport(accessToken, accountId, reportName);
const reportJobIds = createReportResponse.rval.values.map(item => Number(item.reportDefinition.reportJobId));
// Wait 10 seconds for report generation to complete
Utilities.sleep(10000);
reportJobIds.forEach(reportJobId => {
// Retrieving Reports
const downloadResponse = yahooAdDownloadReport(accessToken, accountId, reportJobId);
downloadResponse.forEach(report => {
let d = new Date()
d.setDate(d.getDate() - 1);
let target_row = ss.getRange(ss.getMaxRows(), 2).getNextDataCell(SpreadsheetApp.Direction.UP).getRow() + 1;
ss.getRange(target_row, 1).setValue(report.campaignName)
ss.getRange(target_row, 2).setValue(d)
ss.getRange(target_row, 3).setValue(report.clicks)
ss.getRange(target_row, 4).setValue(report.conversions)
ss.getRange(target_row, 5).setValue(report.cost)
ss.getRange(target_row, 6).setValue(report.imps)
ss.getRange(target_row, 7).setValue(report.clickRate)
ss.getRange(target_row, 8).setValue(report.cpc)
ss.getRange(target_row, 9).setValue(report.cvRate)
ss.getRange(target_row, 10).setValue(report.cvValue)
});
});
}
// Access token acquisition
function yahooAdLogin(clientId, clientSecret, refreshToken) {
const url = "https://biz-oauth.yahoo.co.jp/oauth/v1/token";
const httpResponse = UrlFetchApp.fetch(url, {
method: 'get',
payload: {
grant_type: "refresh_token",
client_id: clientId,
client_secret: clientSecret,
refresh_token: refreshToken,
},
});
const status = httpResponse.getResponseCode();
if (status !== 200) {
throw 'HttpRequestError';
}
const response = JSON.parse(httpResponse.getContentText());
return response;
}
// API calls to generate ad reports
function yahooAdCreateReport(accessToken, accountId, reportName) {
const url = "https://ads-search.yahooapis.jp/api/v7/ReportDefinitionService/add";
const httpResponse = UrlFetchApp.fetch(url, {
method: 'post',
// muteHttpExceptions : true,
contentType: 'application/json',
headers: {
'Authorization': `Bearer ${accessToken}`,
},
payload: JSON.stringify({
accountId:accountId, //修正点
operand: [
{
fields: [
// "DAY" // Required for separate dates.
"CAMPAIGN_NAME", // CAMPAIGN NAME
"CAMPAIGN_START_DATE", // CAMPAIGN START DATE
"CLICKS",
"CONVERSIONS",
"COST",
"IMPS",
"CLICK_RATE",
"AVG_CPC",
"CONV_RATE",
"CONV_VALUE",
],
reportCompressType : "NONE",
reportDateRangeType : "YESTERDAY",
reportDownloadEncode: "UTF8",
reportDownloadFormat: "CSV",
reportIncludeDeleted: "TRUE",
reportLanguage: "JA",
reportName: reportName,
reportType: "CAMPAIGN"
}
],
}),
});
const status = httpResponse.getResponseCode();
if (status !== 200) {
throw 'HttpRequestError';
}
const response = JSON.parse(httpResponse.getContentText());
return response;
}
// Function to retrieve ad reports and parse results
function yahooAdDownloadReport(accessToken, accountId, reportJobId) {
const url = "https://ads-search.yahooapis.jp/api/v7/ReportDefinitionService/download";
const httpResponse = UrlFetchApp.fetch(url, {
method: 'post',
contentType: 'application/json',
headers: {
'Authorization': `Bearer ${accessToken}`,
},
payload: JSON.stringify({
accountId,
reportJobId,
}),
});
const status = httpResponse.getResponseCode();
if (status !== 200) {
throw 'HttpRequestError';
}
const response = httpResponse.getContentText();
const lines = response.split('n');
if (lines.length === 3) {
return [];
}
return lines.slice(1, -2).map((line) => {
const data = line.split(',');
return {
campaignName: String(data[0]),
date : String(data[1]),
clicks : Number(data[2]),
conversions : Number(data[3]),
cost : Number(data[4]),
imps : Number(data[5]),
clickRate : Number(data[6]),
cpc : Number(data[7]),
cvRate : Number(data[8]),
cvValue : Number(data[9]),
};
});
}
Establishment of Acquisition Period
Change the value of reportDateRangeType. 引用元
TODAY | |
YESTERDAY | |
LAST_7_DAYS | Last Monday through Sunday |
LAST_14_DAYS | Last 14 days excluding today |
LAST_30_DAYS | Last 30 days excluding today |
LAST_WEEK | Last Monday through Sunday |
LAST_BUSINESS_WEEK | Last 5 business days from last Monday |
THIS_MONTH | The current month including today |
LAST_MONTH | |
ALL_TIME | All available time periods |
Method of acquisition
The “main" command is used to retrieve the previous day’s data.
Discussion
New Comments
No comments yet. Be the first one!